簡介
由於想找個 UI 的 Framework 套入,並可以支援 Vue3 和 TypeScript ,最後就找到 Naive UI 的套件,那也順便看了一下其它人是如何使用該套件的,就找到了 Naive UI Admin 這個官方分享的免費第三方樣版。
其中就看了一下該樣版是如何把 Axios 套入並使用,也一拼將其整理一下,並試著實作。
開發
安裝 Axios
透過喜歡的方式進行安裝 Axios
1 2 3
| npm install axios
pnpm install axios
|
封裝 Axios
建立 ./src/utils/http/axios
的資料夾,用來存放 Axios 調用的相關程式
建立常用的 Utils
建立 ./src/utils/index.ts
1 2 3 4 5 6 7 8 9 10
| import { isObject } from '@vueuse/core'
export function deepMerge<T = any>(src: any = {}, target: any = {}): T { let key: string for (key in target) src[key] = isObject(src[key]) ? deepMerge(src[key], target[key]) : (src[key] = target[key])
return src }
|
建立可讀性的 Enums
建立 ./src/enums/httpEnum.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
|
export enum RequestEnum { GET = 'GET', POST = 'POST', PATCH = 'PATCH', PUT = 'PUT', DELETE = 'DELETE', }
export enum ContentTypeEnum {
JSON = 'application/json;charset=UTF-8',
TEXT = 'text/plain;charset=UTF-8',
FORM_URLENCODED = 'application/x-www-form-urlencoded;charset=UTF-8',
FORM_DATA = 'multipart/form-data;charset=UTF-8', }
|
建立 Types 聲明
建立 ./src/utils/http/axios/types.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import { AxiosRequestConfig } from 'axios';
export interface CreateAxiosOptions extends AxiosRequestConfig { requestOptions?: RequestOptions; }
export interface RequestOptions { }
export interface Result<T = any> { code: number; type?: 'success' | 'error' | 'warning'; message: string; result?: T; }
|
透過 Class 封裝 Axios 實例
建立 ./src/utils/http/axios/Axios.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| import type { AxiosInstance, AxiosRequestConfig, AxiosResponse } from 'axios' import axios from 'axios' import type { CreateAxiosOptions, RequestOptions, Result } from './types' import { deepMerge } from '~/utils'
export class LKAxios { private axiosInstance: AxiosInstance private options: CreateAxiosOptions constructor(options: CreateAxiosOptions) { this.options = options this.axiosInstance = axios.create(options) }
request<T = any>(config: AxiosRequestConfig, options?: RequestOptions): Promise<T> { const conf: CreateAxiosOptions = deepMerge({}, config) const { requestOptions } = this.options const opt: RequestOptions = Object.assign({}, requestOptions, options) conf.requestOptions = opt
return new Promise((resolve, reject) => { this.axiosInstance .request<any, AxiosResponse<Result>>(conf) .then((res: AxiosResponse<Result>) => { resolve(res as unknown as Promise<T>) }) }) } }
|
建立調用 Axios 入口
建立 ./src/utils/http/axios/index.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import { ContentTypeEnum } from '~/enums/httpEnum' import { deepMerge } from '~/utils' import { LKAxios } from './Axios' import type { CreateAxiosOptions } from './types'
function createAxios(opt?: Partial<CreateAxiosOptions>) { return new LKAxios( deepMerge({ baseURL: '', timeout: 10 * 1000, headers: { 'Content-Type': ContentTypeEnum.JSON }, requestOptions: { } }, opt || {})) }
export const http = createAxios()
|
測試
在任意的 .vue
檔案中
1 2 3 4 5 6 7 8
| <script setup lang="ts"> import { http } from '~/utils/http/axios'
http.request({ url: '/api/v1/test' }).then((res) => { console.log(res.data) }) </script>
|
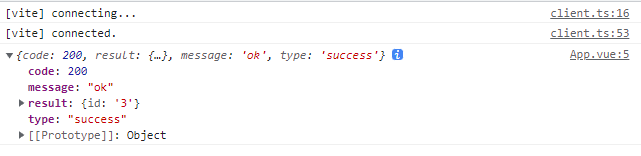
參考
Naive UI Third-Party Libraries
Naive UI Admin
Axios
Vitesse
Sample Code Download